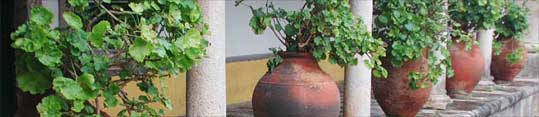
Hide/Show Div page content (div, p, input, etc.)
"How do I make items on my page appear or disappear?
Here is a basic example where 2 items appear on the page and we will make them "Hide" or "Show" by changing the class associated with each.
Note: There are a lot of ways to do this. This example presumes that each element that you are hiding or showing will only ever have 1 class assigned to it - which is not always the case.
Also, if you try to toggle the class of an element that does not have any class assigned to it (DIV3), that element does not hide or show.
See also: Hide/Show by changing style.display
Here's how it works:
- In HTML, all 3 items contain the class "inline"
- The CSS for this is
- The link to toggle the display of each div calls the javascript function toggleDisplay(item) where item is the id of the item on the page that we want to hide or show.
- The javascript function toggleDisplay(item) verifies that the item exists on the page, that the item supports the class attribute, and then changes the class based on the current setting. If it is currently "inline", it changes it to "hidden". If no class is found, it adds "inline" so that subsequent clicks can hide it.
<div id="div1" class="inline">
<div>DIV1</div>
</div>
<div id="div2" class="inline">
<div>DIV2</div>
</div>
<div>DIV1</div>
</div>
<div id="div2" class="inline">
<div>DIV2</div>
</div>
.hidden {display:none;}
.inline {display:inline;}
.inline {display:inline;}
<a href="javascript:void(0);" onclick="toggleDisplay('div1');">DIV1</a>
function toggleDisplay(item) {
if (document.getElementById(item)) {
if (document.getElementById(item).className) {
switch (document.getElementById(item).className) {
case "inline":
document.getElementById(item).className = 'hidden';
break;
default:
document.getElementById(item).className = 'inline';
}
}
}
}
if (document.getElementById(item)) {
if (document.getElementById(item).className) {
switch (document.getElementById(item).className) {
case "inline":
document.getElementById(item).className = 'hidden';
break;
default:
document.getElementById(item).className = 'inline';
}
}
}
}